- Jan 17, 2014
- 4
- 2
- 9
Hello guys. I've built an automated door using an arduino controller and a clock for controlling it. I converted a former pig house for the hens a while ago, but it has the disadvantage that it is quite small. I wanted to let the chicks roam free in the daylight and still be protected at night and I had some spare time to do it. The materials used are pretty basic, I've used some printed parts form my 3D printer, but they are nothing so much special and I think you can find a way to substitute them.
So here is what I did.
I started with the electrical stuff. Here is the parts list along with estimated costs:
arduino http://dx.com/p/arduino-nano-v3-0-81877#.UtmuVvT8KQ8 10-20$
motor driver http://dx.com/p/l298n-stepper-motor-driver-controller-board-for-arduino-120542#.UtmtRvT8KQ8 6$
clock http://dx.com/p/pcf8563-rtc-board-for-raspberry-pi-real-time-clock-module-blue-281614#.Utmtw_T8KQ8 6$
geared motor ~ 5-10$
wires-connectors etc ~ 10$
2 end stops - 1$
aluminum rails - 10$
printed parts - reel and rail holders
total - 50-60$
Basically I just needed to wire them together. The clock and motor driver wiring is pretty straightforward, the buttons are using a 10K pullup resistor and their normal state is closed. When they are clicked they open the circuit which is better for the emergency case when one of the buttons disconnects (maybe the rooster ate it). Then the program will think the button is pressed and the motor will not run without stopping. If you want I can provide you with the exact wiring on my setup, but you can use other parts and modify slightly the program and it will still work. Also you can check out the following picture:

Note that I used a geared motor, as it can lift a not-so-light door and also keep it in the up position when no power is applied to the motor. The reel is printed, you don't really care of its diameter, you just want to be able to roll the whole cord on it and mount it well to the motor.
This is the source code I am using:
#include <Wire.h>
#include <Rtc_Pcf8563.h>
#include <Time.h>
#define POS_UNKNOWN 0
#define POS_TOP 1
#define POS_BOT 2
int MOTOR_A_EN_PIN = 6;
int MOTOR_A_IN1_PIN = 7;
int MOTOR_A_IN2_PIN = 8;
int MOTOR_A_SPEED = 255;
int TOP_BUT_PIN = 9;
int BOT_BUT_PIN = 10;
//init the real time clock
Rtc_Pcf8563 rtc;
int pos = POS_UNKNOWN;
int desiredPos = POS_BOT;
// Time represents just hour/min and time = hour * 60 + min
int raiseTime = 430; // 7:10
int lowerTime = 1130; // 18:50
//int raiseTime = 678; // 7:10
//int lowerTime = 679; // 18:50
void setup()
{
Serial.begin(9600);
pinMode(MOTOR_A_EN_PIN, OUTPUT);
pinMode(MOTOR_A_IN1_PIN, OUTPUT);
pinMode(MOTOR_A_IN2_PIN, OUTPUT);
pinMode(TOP_BUT_PIN, INPUT);
pinMode(BOT_BUT_PIN, INPUT);
delay(1000);
//DO NOT UPDATE TIME ANYMORE
// //clear out the registers
// rtc.initClock();
// //set a time to start with.
// //day, weekday, month, century(1=1900, 0=2000), year(0-99)
// rtc.setDate(14, 1, 1, 0, 14);
// //hr, min, sec
// rtc.setTime(3, 54, 0);
}
void loop()
{
desiredPos = calcDesiredPos();
if (desiredPos == pos) {
delay(1000);
return;
}
syncTime();
if (desiredPos == POS_BOT) {
runMotor(false);
while (!reachedBot()) {
delay(50);
}
stopMotor();
pos = POS_BOT;
} else if (desiredPos == POS_TOP) {
runMotor(true);
while (!reachedTop()) {
delay(50);
}
stopMotor();
pos = POS_TOP;
}
}
void runMotor(boolean dir) {
digitalWrite(MOTOR_A_IN1_PIN, dir ? HIGH : LOW);
digitalWrite(MOTOR_A_IN2_PIN, dir ? LOW : HIGH);
digitalWrite(MOTOR_A_EN_PIN, MOTOR_A_SPEED);
}
void stopMotor() {
digitalWrite(MOTOR_A_IN1_PIN, LOW);
digitalWrite(MOTOR_A_IN2_PIN, LOW);
digitalWrite(MOTOR_A_EN_PIN, 0);
}
boolean reachedTop() {
return digitalRead(TOP_BUT_PIN) == LOW;
}
boolean reachedBot() {
return digitalRead(BOT_BUT_PIN) == LOW;
}
int calcDesiredPos() {
int timeNow = hour() * 60 + minute();
if (raiseTime <= timeNow && timeNow < lowerTime) {
return POS_TOP;
}
return POS_BOT;
}
void syncTime() {
setTime(rtc.getHour(), rtc.getMinute(), rtc.getSecond(),
rtc.getDay(), rtc.getMonth(), rtc.getYear());
}
The parts were assembled into a former cheese container (I happen to like them a lot as they provide more or less safe environment and also a mounting option which you will see below.
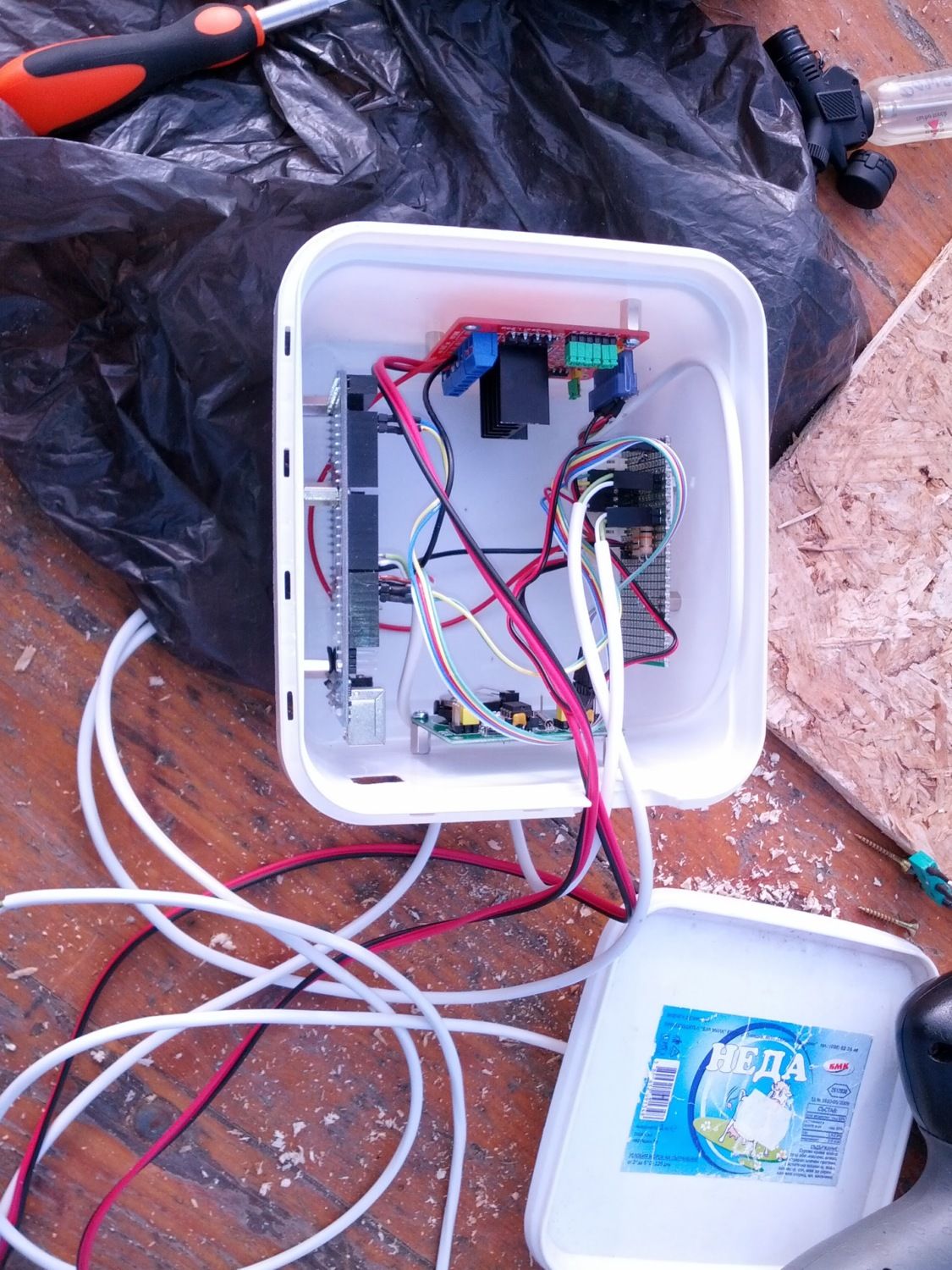
I bought some extruded aluminum in an E shape. It has two channels which is handy as I used one of them to secure it to the frame of the door and the other to slide the door in.

They were 1 meter long so I've cut them in half. I didn't want to use 2 rods as you can still let the door stick out of it by 5 cm for example. Also I will not tolerate fat chicks.
For the mounting I've printed some parts which connect to the rails with bolts and have another hole for screwing to the door frame. I cut the door approximately 30x30cm in the frame where I mounted the rails with the door plate inside. I've also cut a hole for the motor reel.

I really didn't want the motor to move so you can see the result. Not that pretty, but effective I hope.
For the plate I intended to use acrylic sheet, but then found some eternit plates lying around that I shaped using pliers. I think the acrylic would be a better way to go as the door became a bit heavy.

On the top and bottom I've added the limit switches and basically it was done. On the following picture, the door is reversed.

I've also moved the upper limit switch so it opens more and its opened quite a lot now as even the rooster has no trouble going in and out. Here is an old picture of him laying an egg:

And then the phone battery was dead and I couldn't take any more pictures. The mounted pictures are TBD. If you like it I can do a video of it in action.
It took me about 2 days to finish, but I enjoyed doing it. When you think of the cost its not too little. But the good thing is that it's extensible. You can easily add for example a water sourse with a solenoid for always fresh water (remember you can reuse the clock). Or maybe opening windows to correct temperature. You can basically do anything you imagine.
So here is what I did.
I started with the electrical stuff. Here is the parts list along with estimated costs:
arduino http://dx.com/p/arduino-nano-v3-0-81877#.UtmuVvT8KQ8 10-20$
motor driver http://dx.com/p/l298n-stepper-motor-driver-controller-board-for-arduino-120542#.UtmtRvT8KQ8 6$
clock http://dx.com/p/pcf8563-rtc-board-for-raspberry-pi-real-time-clock-module-blue-281614#.Utmtw_T8KQ8 6$
geared motor ~ 5-10$
wires-connectors etc ~ 10$
2 end stops - 1$
aluminum rails - 10$
printed parts - reel and rail holders
total - 50-60$
Basically I just needed to wire them together. The clock and motor driver wiring is pretty straightforward, the buttons are using a 10K pullup resistor and their normal state is closed. When they are clicked they open the circuit which is better for the emergency case when one of the buttons disconnects (maybe the rooster ate it). Then the program will think the button is pressed and the motor will not run without stopping. If you want I can provide you with the exact wiring on my setup, but you can use other parts and modify slightly the program and it will still work. Also you can check out the following picture:
Note that I used a geared motor, as it can lift a not-so-light door and also keep it in the up position when no power is applied to the motor. The reel is printed, you don't really care of its diameter, you just want to be able to roll the whole cord on it and mount it well to the motor.
This is the source code I am using:
#include <Wire.h>
#include <Rtc_Pcf8563.h>
#include <Time.h>
#define POS_UNKNOWN 0
#define POS_TOP 1
#define POS_BOT 2
int MOTOR_A_EN_PIN = 6;
int MOTOR_A_IN1_PIN = 7;
int MOTOR_A_IN2_PIN = 8;
int MOTOR_A_SPEED = 255;
int TOP_BUT_PIN = 9;
int BOT_BUT_PIN = 10;
//init the real time clock
Rtc_Pcf8563 rtc;
int pos = POS_UNKNOWN;
int desiredPos = POS_BOT;
// Time represents just hour/min and time = hour * 60 + min
int raiseTime = 430; // 7:10
int lowerTime = 1130; // 18:50
//int raiseTime = 678; // 7:10
//int lowerTime = 679; // 18:50
void setup()
{
Serial.begin(9600);
pinMode(MOTOR_A_EN_PIN, OUTPUT);
pinMode(MOTOR_A_IN1_PIN, OUTPUT);
pinMode(MOTOR_A_IN2_PIN, OUTPUT);
pinMode(TOP_BUT_PIN, INPUT);
pinMode(BOT_BUT_PIN, INPUT);
delay(1000);
//DO NOT UPDATE TIME ANYMORE
// //clear out the registers
// rtc.initClock();
// //set a time to start with.
// //day, weekday, month, century(1=1900, 0=2000), year(0-99)
// rtc.setDate(14, 1, 1, 0, 14);
// //hr, min, sec
// rtc.setTime(3, 54, 0);
}
void loop()
{
desiredPos = calcDesiredPos();
if (desiredPos == pos) {
delay(1000);
return;
}
syncTime();
if (desiredPos == POS_BOT) {
runMotor(false);
while (!reachedBot()) {
delay(50);
}
stopMotor();
pos = POS_BOT;
} else if (desiredPos == POS_TOP) {
runMotor(true);
while (!reachedTop()) {
delay(50);
}
stopMotor();
pos = POS_TOP;
}
}
void runMotor(boolean dir) {
digitalWrite(MOTOR_A_IN1_PIN, dir ? HIGH : LOW);
digitalWrite(MOTOR_A_IN2_PIN, dir ? LOW : HIGH);
digitalWrite(MOTOR_A_EN_PIN, MOTOR_A_SPEED);
}
void stopMotor() {
digitalWrite(MOTOR_A_IN1_PIN, LOW);
digitalWrite(MOTOR_A_IN2_PIN, LOW);
digitalWrite(MOTOR_A_EN_PIN, 0);
}
boolean reachedTop() {
return digitalRead(TOP_BUT_PIN) == LOW;
}
boolean reachedBot() {
return digitalRead(BOT_BUT_PIN) == LOW;
}
int calcDesiredPos() {
int timeNow = hour() * 60 + minute();
if (raiseTime <= timeNow && timeNow < lowerTime) {
return POS_TOP;
}
return POS_BOT;
}
void syncTime() {
setTime(rtc.getHour(), rtc.getMinute(), rtc.getSecond(),
rtc.getDay(), rtc.getMonth(), rtc.getYear());
}
The parts were assembled into a former cheese container (I happen to like them a lot as they provide more or less safe environment and also a mounting option which you will see below.
I bought some extruded aluminum in an E shape. It has two channels which is handy as I used one of them to secure it to the frame of the door and the other to slide the door in.
They were 1 meter long so I've cut them in half. I didn't want to use 2 rods as you can still let the door stick out of it by 5 cm for example. Also I will not tolerate fat chicks.
For the mounting I've printed some parts which connect to the rails with bolts and have another hole for screwing to the door frame. I cut the door approximately 30x30cm in the frame where I mounted the rails with the door plate inside. I've also cut a hole for the motor reel.
I really didn't want the motor to move so you can see the result. Not that pretty, but effective I hope.
For the plate I intended to use acrylic sheet, but then found some eternit plates lying around that I shaped using pliers. I think the acrylic would be a better way to go as the door became a bit heavy.
On the top and bottom I've added the limit switches and basically it was done. On the following picture, the door is reversed.
I've also moved the upper limit switch so it opens more and its opened quite a lot now as even the rooster has no trouble going in and out. Here is an old picture of him laying an egg:
And then the phone battery was dead and I couldn't take any more pictures. The mounted pictures are TBD. If you like it I can do a video of it in action.
It took me about 2 days to finish, but I enjoyed doing it. When you think of the cost its not too little. But the good thing is that it's extensible. You can easily add for example a water sourse with a solenoid for always fresh water (remember you can reuse the clock). Or maybe opening windows to correct temperature. You can basically do anything you imagine.