This thread is to show how I did my coop automation. I'm going to start as basic as I can. I have tried Arduino's and ESP8266 and other control devices and finally settled in with the Raspberry Pi 3B as my control of choice. The programming is in Python which to me is a bit easier to read than C which you must use for the Arduino and other devices that support Arduino code.
Some things to know up front, I do all my programming on a Linux PC. If you connect a keyboard, mouse and monitor to the Raspberry Pi you can program directly on the Rpi (Raspberry Pi). The Rpi uses a Linux Operating System, nothing to be afraid of, it just works better. The Rpi needs a connection to the Internet at bootup to get the current date and time, the Rpi doesn't have an onboard real time clock or battery/capacitor backup for date and time. The Rpi is a SBC (Single Board Computer), yes it's a fully functioning PC with USB ports, HDMI monitor connection and 40 pins that are used for I/O (Input/Output), these are use to connect the Rpi to the real world. You use the I/O to run your motor, turn lights on and off, get input from limit switches etc. The Rpi has some special pins, I2C or One Wire which can connect to things like temperature sensors (you can connect more than one as they have a unique address). The Rpi I/O is 3.3vdc so you need to be careful and select 3vdc relays, while 5v relays may work it's better to use the 3v versions.
Python is a programming language not the snake. The most simple program you could run is howdy.py
The first line is the shebang line that tells the system what to use to run the code.
The second line is a print statement that does what it looks like it should do.
To run the program the executable permission must be set. The easy way is to right click on the file in the file manager and select permissions then check off executable.
Then open a terminal in the same directory and type in ./howdy.py the ./ is needed otherwise the file will not be found.
You can also test snippets of code in a terminal without creating a file and I do that all the time to just test syntax and see my results. To start a Python session just type in python3 and press enter. Then you can test your code snippet.
Time to start my day,next up is the event loop and you will need one...
Got ahead of myself...
JT
Some things to know up front, I do all my programming on a Linux PC. If you connect a keyboard, mouse and monitor to the Raspberry Pi you can program directly on the Rpi (Raspberry Pi). The Rpi uses a Linux Operating System, nothing to be afraid of, it just works better. The Rpi needs a connection to the Internet at bootup to get the current date and time, the Rpi doesn't have an onboard real time clock or battery/capacitor backup for date and time. The Rpi is a SBC (Single Board Computer), yes it's a fully functioning PC with USB ports, HDMI monitor connection and 40 pins that are used for I/O (Input/Output), these are use to connect the Rpi to the real world. You use the I/O to run your motor, turn lights on and off, get input from limit switches etc. The Rpi has some special pins, I2C or One Wire which can connect to things like temperature sensors (you can connect more than one as they have a unique address). The Rpi I/O is 3.3vdc so you need to be careful and select 3vdc relays, while 5v relays may work it's better to use the 3v versions.
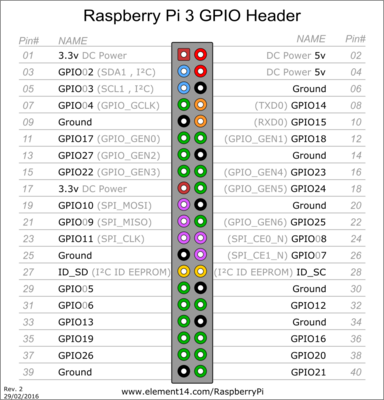
Python is a programming language not the snake. The most simple program you could run is howdy.py
Python:
#!/usr/bin/python3
print("Howdy")
The first line is the shebang line that tells the system what to use to run the code.
The second line is a print statement that does what it looks like it should do.
To run the program the executable permission must be set. The easy way is to right click on the file in the file manager and select permissions then check off executable.
Then open a terminal in the same directory and type in ./howdy.py the ./ is needed otherwise the file will not be found.
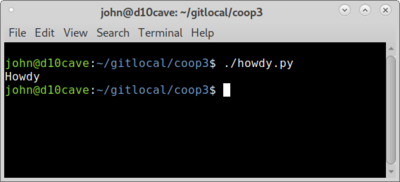
You can also test snippets of code in a terminal without creating a file and I do that all the time to just test syntax and see my results. To start a Python session just type in python3 and press enter. Then you can test your code snippet.
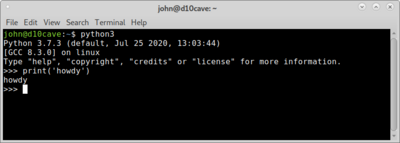
Time to start my day,
Got ahead of myself...
JT
Last edited: